2025 Author: Howard Calhoun | [email protected]. Last modified: 2025-01-24 13:10
In this article, the scanf() function is considered in a general form without reference to a specific standard, so data from any C99, C11, C++11, C++14 standards is included here. Perhaps, in some standards, the function works with differences from the material presented in the article.
scanf C function - description
scanf() is a function located in the stdio.h(C) and cstdio(C++) header files, also known as formatted program input. scanf reads characters from the standard input stream (stdin) and converts them according to the format, then writes them to the specified variables. Format - means that the data is converted to a certain form upon receipt. Thus, the scanf C function is described:
scanf("%format", &variable1[, &variable2, […]), where variables are passed as addresses. The reason for this way of passing variables to a function is obvious: as a result of work, it returns a value indicating the presence of errors, sothe only way to change the values of variables is by passing by address. Also, thanks to this method, the function can process data of any type.
Some programmers refer to functions like scanf() or printf() as procedures because of the analogy with other languages.
Scanf allows input of all basic language types: char, int, float, string, etc. In the case of variables of type string, there is no need to specify the address sign - "&", since the variable of type string is an array, and its name is the address of the first element of the array in the computer's memory.
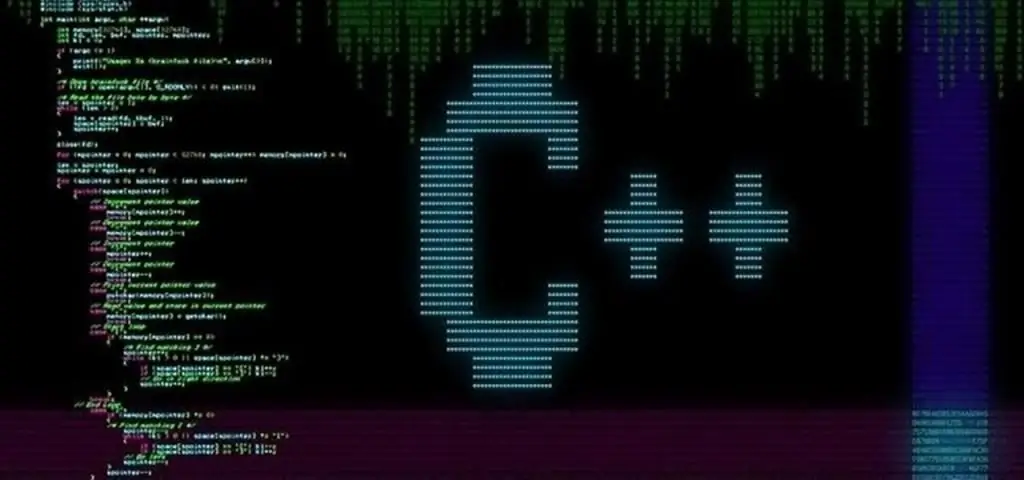
Data entry format or control string
Start by looking at the scanf C function example from the description.
include int main() { int x; while (scanf("%d", &x)==1) printf("%d\n", x); return 0; //requirement for linux systems }
The input format consists of the following four parameters: %[width][modifiers] type. In this case, the "%" sign and the type are mandatory parameters. That is, the minimum format look like this: “%s”, “%d” and so on.
In general, the characters that make up the format string are divided into:
- format specifiers - anything starting with %;
- separating or space characters - they are space, tab(t), newline(n);
- characters other than whitespace.
Function may not be safe.
Use scanf_s() instead of scanf().
(message from Visual Studio)
Type, or format specifiers, or conversion characters, or control characters
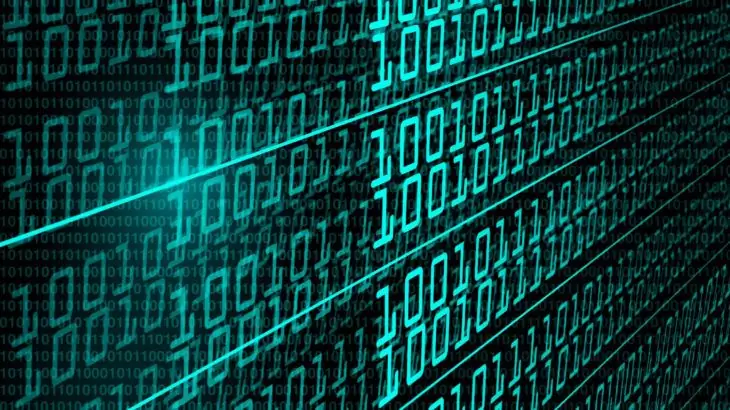
A scanf C declaration must contain at least a format specifier, which is specified at the end of expressions beginning with "%". It tells the program the type of data to expect when entering, usually from the keyboard. List of all format specifiers in the table below.
Type | Meaning | |
1 | %c | The program is waiting for a character input. The variable to be written must be of the character type char. |
2 | %d | The program expects input of a decimal number of integer type. The variable must be of type int. |
3 | %i |
The program expects input of a decimal number of integer type. The variable must be of type int. |
4 | %e, %E | The program expects to enter a floating point (comma) number in exponential form. The variable must be of type float. |
5 | %f | The program expects a floating point number (comma). The variable must be of type float. |
6 | %g, %G | The program expects a floating point number (comma). The variable must be of type float. |
7 | %a | The program expects a floating point number (comma). The variable must be of type float. |
8 | %o | The program expects an octal number. The variable must be of type int. |
9 | %s | The program is waiting for a string to be entered. A string is a set of any characters up to the first separator character encountered. The variable must be of type string. |
10 | %x, %X | The program is waiting for a hexadecimal number. The variable must be of type int. |
11 | %p | Variable expects pointer input. The variable must be of pointer type. |
12 | %n | Writes an integer value to the variable, equal to the number of characters read so far by the scanf function. |
13 | %u | The program reads an unsigned integer. The variable type must be unsigned integer. |
14 | %b | The program is waiting for a binary number. The variable must be of type int. |
15 | % |
Scanned character set. The program is waiting for characters to be entered.from the limited pool specified between square brackets. scanf will work as long as there are characters from the specified set on the input stream. |
16 | %% | Sign "%". |
Characters in the format string
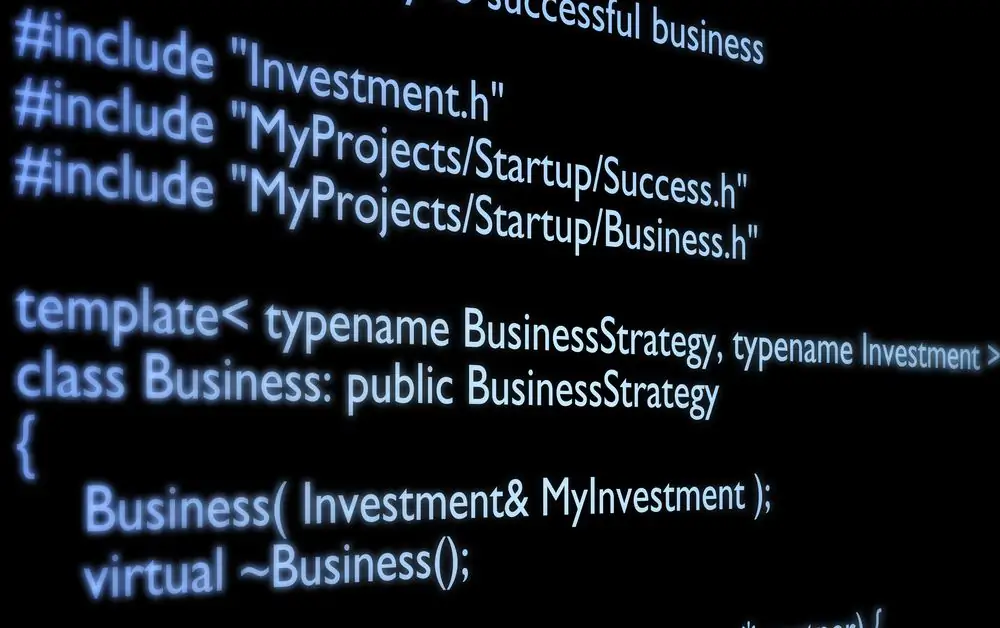
Star symbol ()
The asterisk () is a flag indicating that the assignment operation should be suppressed. An asterisk is placed immediately after the "%" sign. For example,
scanf("%d%c%d", &x, &y); //ignore character between two integers. scanf("%s%d%s", str, str2); //ignore integer between two strings.
That is, if you enter the line "45-20" in the console, the program will do the following:
- Variable "x" will be assigned the value 45.
- Variable "y" will be assigned the value 20.
- And the minus sign (dash) "-" will be ignored thanks to "%c".
Width (or field width)
This is an integer between the "%" sign and the format specifier that specifies the maximum number of characters to read in the current read operation.
scanf("%20s", str); //read the first 20 characters from the input stream
There are a few important things to keep in mind:
- scanf will terminate if it encounters a separator character, even if it didn't count 20 characters.
- If more than 20 characters are input, only the first 20 characters will be written to str.
Modifierstype (or precision)
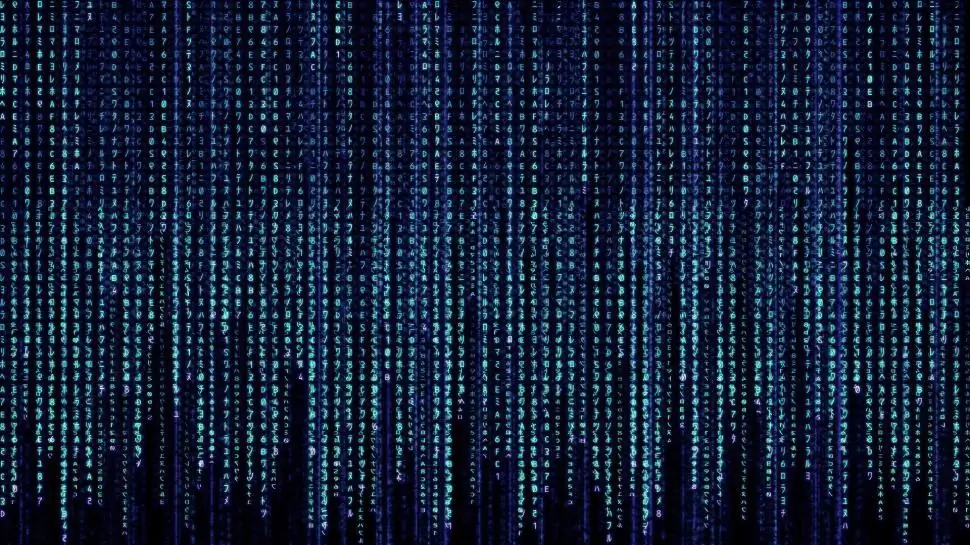
These are special flags that modify the type of data expected for input. The flag is specified to the left of the type specifier:
- L or l (small L) When "l" is used with the specifiers d, i, o, u, x, the flag tells the program that long int input is expected. When using "l" with the e or f specifier, the flag tells the program that it should expect a double value. The use of "L" tells the program that a long double is expected. The use of "l" with the "c" and "s" specifiers tells the program that two-byte characters like wchar_t are expected. For example, "%lc", "%ls", "%l[asd]".
- h is a flag indicating the short type.
- hh - indicates that the variable is a pointer to a signed char or unsigned char value. The flag can be used with the specifiers d, i, o, u, x, n.
- ll (two small L's) - indicates that the variable is a pointer to a value of type signed long long int or unsigned long long int. The flag is used with specifiers: d, i, o, u, x, n.
- j - indicates that the variable is a pointer to the intmax_t or uintmax_t type from the stdint.h header file. Used with specifiers: d, i, o, u, x, n.
- z - indicates that the variable is a pointer to the size_t type, the definition of which is in stddef.h. Used with specifiers: d, i, o, u, x, n.
- t - indicates that the variable is a pointer to the ptrdiff_t type. Definition onthis type is in stddef.h. Used with specifiers: d, i, o, u, x, n.
More clearly, the picture with modifiers can be represented as a table. Such a description of scanf C for programmers will be clearer.
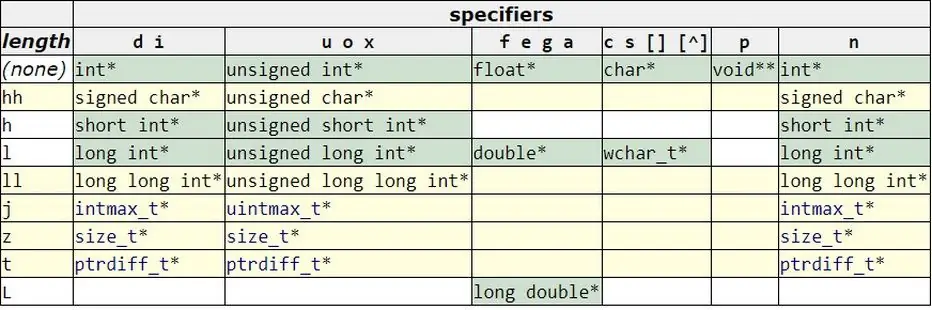
Other characters
Any characters encountered in the format will be discarded. At the same time, it should be noted that the presence of whitespace or separator characters (newline, space, tab) in the control string can lead to different behavior of the function. In one version, scanf() will read without saving any number of separators until it encounters a character other than the separator, and in another version, spaces (only they) do not play a role and the expression "%d + %d" is equivalent to "% d+%d".
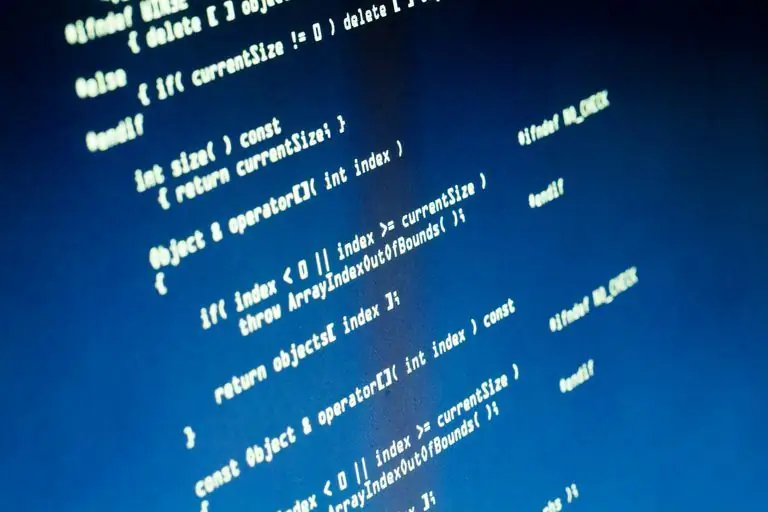
Examples
Let's look at some examples to help you think and better understand how the function works.
scanf("%3s", str); //if you enter the string "1d2s3d1;3" in the console, only "1d2" will be written to str scanf("%dminus%d", &x, &y); //minus characters between two numbers will be discarded scanf("%5[0-9]", str); // characters will be entered into str until there are 5 characters and the characters are numbers from 0 to 9. scanf("%lf", &d); //expect double input scanf("%hd", &x); //expected number of type short scanf("%hu", &y); //expect unsigned number short scanf("lx", &z); //expected number of type long int
FromThe examples below show how the expected number changes using different symbols.
scanf C - description for beginners
This section will be useful for beginners. Often you need to have at hand not so much a complete description of scanf C as the details of how the function works.
- This feature is somewhat obsolete. There are several different implementations in libraries of different versions. For example, the improved scanf S C function, a description of which can be found on the microsoft website.
- The number of specifiers in the format must match the number of arguments passed to the function.
- Input stream elements must be separated only by separator characters: space, tab, newline. Comma, semicolon, period, etc. - these characters are not separators for the scanf() function.
- If scanf encounters a separator character, input will be stopped. If there is more than one variable to read, then scanf will move on to reading the next variable.
- The slightest inconsistency in the input data format leads to unpredictable results of the program. Well, if the program just ends with an error. But often the program continues to work and does it wrong.
- scanf("%20s …", …); If the input stream exceeds 20 characters, then scanf will read the first 20 characters and either abort or move on to reading the next variable, if one is specified. In this case, the next call to scanf will continue reading the input stream from the point where the work of the previous call to scanf stopped. If when reading the first 20characters, a delimiter character is encountered, scanf will abort or continue reading the next variable, even if it did not read 20 characters for the first variable. In this case, all unread characters will be attached to the next variable.
- If the set of scanned characters starts with a "^", then scanf will read the data until it encounters a delimiter character or a character from the set. For example, "%[^A-E1-5]" will read data from the stream until one of the uppercase English characters from A to E or one of the numbers from 1 to 5 is encountered.
- The scanf C function, as described, returns a number equal to the successful number of writes to variables. If scanf writes 3 variables, then the success result of the function will return the number 3. If scanf could not write any variables, then the result will be 0. And, finally, if scanf could not start at all for some reason, the result will be EOF.
- If the scanf() function ended incorrectly. For example, scanf("%d", &x) - a number was expected, but characters were received as input. The next scanf() call will start at the point in the input stream where the previous function call ended. To overcome this problem, it is necessary to get rid of the problem characters. This can be done, for example, by calling scanf("%s"). That is, the function will read a string of characters and throw it away. In this tricky way, you can continue entering the necessary data.
- In some implementations of scanf(), "-" is not allowed in the scanned character set.
- The "%c" specifier reads each character from the stream. That is, it also reads the separator character. To skip the delimiter character and continue reading the desired character, you can use "%1s".
- When using the "c" specifier, it is permissible to use the width "%10c", but then an array of elements of type char must be passed as a variable to the scanf function.
- “%[a-z]” means “all small letters of the English alphabet”, and “%[z-a]” means just 3 characters: ‘z’, ‘a’, ‘-’. In other words, the "-" character means a range only if it is between two characters that are in the correct order. If "-" is at the end of an expression, at the beginning, or in the wrong order of characters on either side of them, then it is just a hyphen character, not a range.
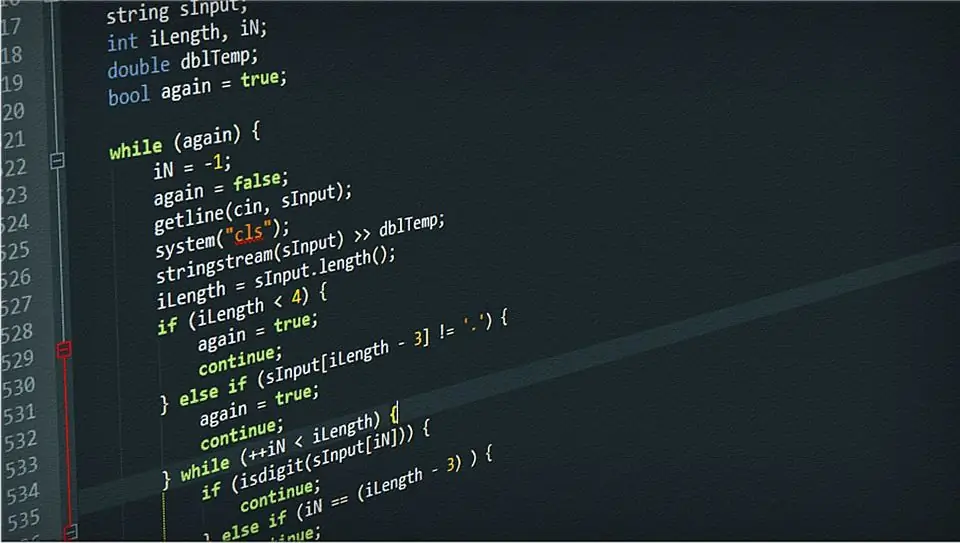
Conclusion
This concludes the description of scanf C. This is a nice handy feature for working in small programs and when using the procedural programming method. However, the main disadvantage is the number of unpredictable errors that can occur when using scanf. Therefore, the description of scanf C when programming is best kept in front of your eyes. In large professional projects, iostreams are used, due to the fact that they have higher-level capabilities, they are better able to catch and handle errors, and also work with significant amounts of information. It should also be noted that the description of scanf C in Russian is available on many network sources, as well as examples of ituse, due to the age of the function. Therefore, if necessary, you can always find the answer on the thematic forums.
Recommended:
Leasing: advantages and disadvantages, main function, classification, tips and tricks
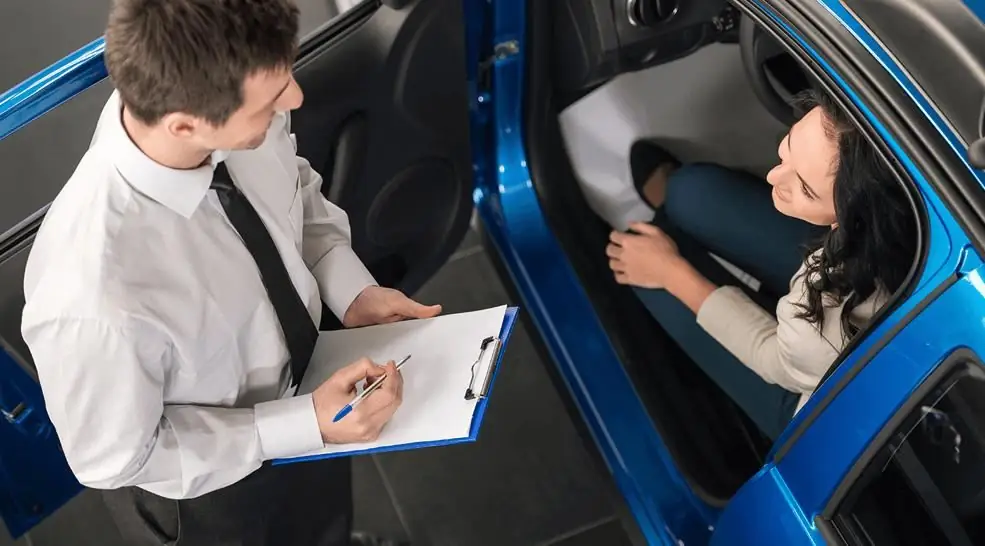
Definition, functions, advantages and disadvantages of leasing as a form of financial activity. General information, classification of leasing relations. Pros and cons of buying a car on lease, as the most common product. Recommendations for choosing a leasing company
The main function of control in management
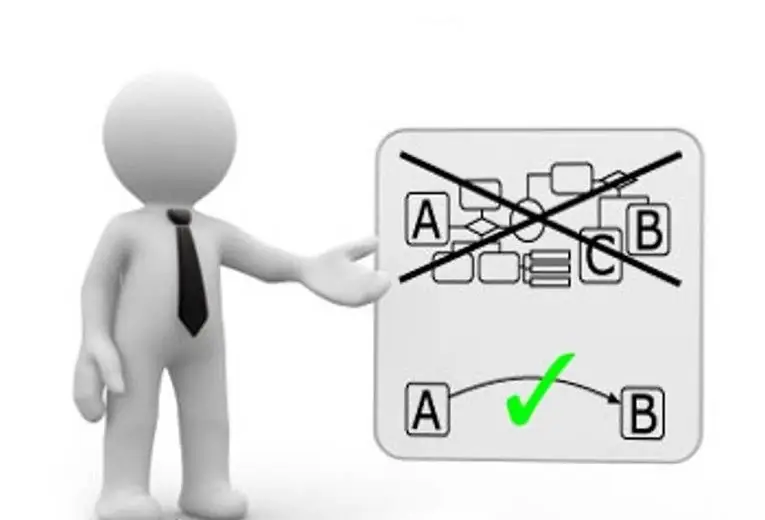
Any leader performs the basic functions of management: planning, organization, motivation, control. Four elements of the control function: defining indicators and methods for measuring results, measuring results, determining whether results are as planned, and corrective actions
Control function of taxes: description and examples
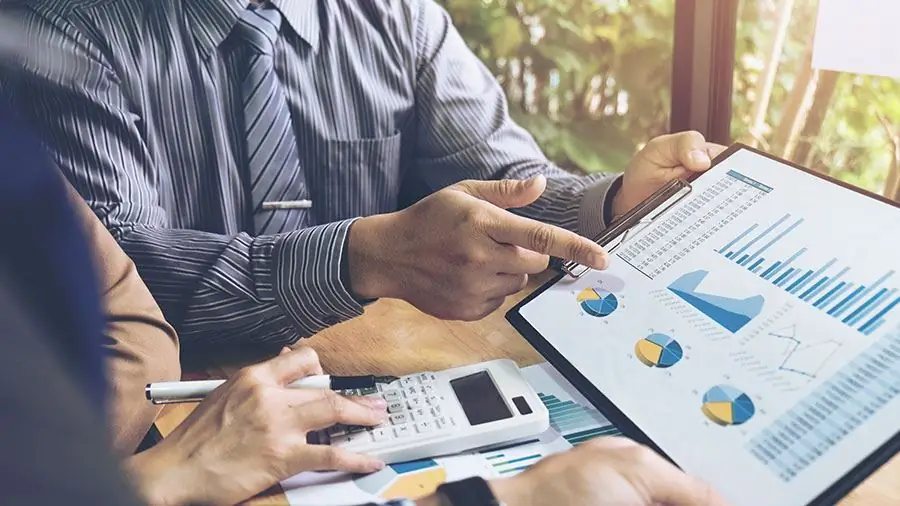
What are taxes? Characteristics and examples of their functions: control, social (redistributive), regulatory, fiscal. What sub-functions stand out here? What is the additional incentive function? What are the control and other functions of the Federal Tax Service?
The tasks of the leader: key responsibilities, requirements, role, function and achievement of the goal
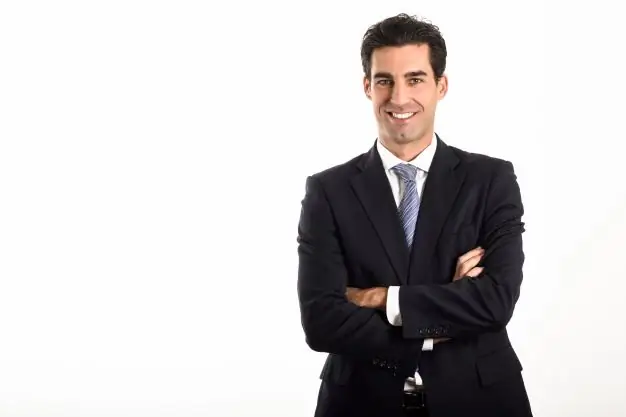
Are you planning a promotion soon? So it's time to get ready for it. What are the challenges leaders face on a daily basis? What does a person need to know who is going to take on the burden of responsibility for other people in the future? Read all about it below
What is physical security? How does it function and what is its purpose?
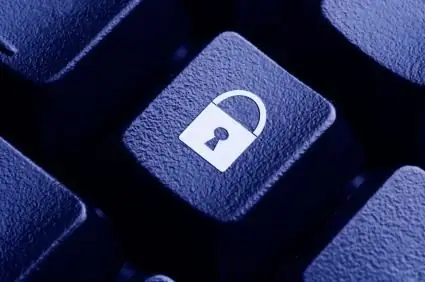
An article about what the task of physical security is, what it is and how it works. The main requirements for personnel in this area are also given